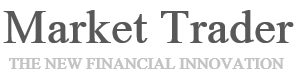
How to Program Your Own Expert Advisor: A Step-by-Step Guide
The Basics of the MQL5 Programming Language
MQL5 is a programming language specifically developed for the MetaTrader platform, allowing the creation of automated trading strategies (Expert Advisors), scripts, and custom indicators. It is based on the syntax of C++ and offers a wide range of functions designed for trading.
Some basic elements of MQL5 include:
- Data Types: MQL5 supports various data types such as
int
(integers),double
(floating-point numbers), andstring
(text strings). - Functions: In MQL5, functions are the core component of any EA. They help structure the code and can be reused.
- Control Structures: Like other programming languages, MQL5 has loops (
for
,while
) and conditional statements (if
,else
) that control the flow of the program.
For an Expert Advisor, specific elements such as OnTick()
and OnInit()
are crucial as they govern the EA’s actions based on market events and initializations.
How to Translate Your Trading Strategy into Code
To turn a trading strategy into an Expert Advisor, you first need to translate the logic of your strategy into clear rules. These rules will form the basis of your code. Here is a general approach:
- Define the entry and exit rules of your strategy: Under what conditions should the EA open a position, and when should it close it?
- Create a list of the indicators you want to use and determine how their signals will be integrated into the code.
- Establish the risk and money management rules: How much capital should be risked per trade, and how will you set the stop-loss and take-profit?
An example of starting the code might look like this:
int OnInit() { // Initialization of the EA return INIT_SUCCEEDED; }
void OnTick() { // Main logic of the EA, // executed on each market tick if (SignalDetected()) { // Open position OrderSend(...); } }
Key Functions and Variables for the EA
When programming an EA, some functions and variables are especially important for making trading decisions and managing positions:
OnInit()
: This function is called once at the start of the EA and is used to initialize variables and settings.OnTick()
: This function is called with every new market tick and contains the main logic of the EA.OrderSend()
: This function is used to send a trading order and open a position.- Global Variables: You can use global variables to store important information, such as the current position or risk level.
iCustom()
: This function allows you to integrate custom indicators into your EA.
Backtesting Your EA Before Going Live
Before deploying your Expert Advisor in live markets, it is important to test it thoroughly. The MetaTrader Strategy Tester is ideal for this purpose. Here is a step-by-step guide to backtesting:
- Open the Strategy Tester in MetaTrader.
- Select your EA from the list of Expert Advisors.
- Set the test parameters: Choose the period, symbol, and time frame you want to test.
- Run the test and analyze the results: Pay particular attention to metrics like the profit factor, win rate, and maximum drawdown.
- Optimize the EA based on the test results and repeat the backtesting until you are satisfied.
Common Mistakes When Programming an EA and How to Avoid Them
When programming an Expert Advisor, common mistakes can occur that lead to unexpected results or losses. Here are some frequent mistakes and tips on how to avoid them:
- Faulty Logic: Ensure that your entry and exit criteria are clearly and precisely defined. Test each part of your code thoroughly.
- Lack of Error Handling: Implement error-handling mechanisms, such as catching order errors, to ensure the EA remains stable during unforeseen situations.
- Failure to Adapt to Market Conditions: A static EA may perform poorly in different market phases. Consider various market conditions and build flexibility into your code.
- Overleveraging: Ensure that your position sizes are not too large and use strict risk management to avoid capital losses.